On your request, as an addition to the how-to: Google Form to PDF (save to Drive and/or send it in email), here is a script to create a new Google Slide from a slide template and save it to PDF or send it in an email.
So, this Google Form script will open a Google Slide presentation and replace the text with the text from the Form, and then it will save it as a new Google Slide presentation. Also, there is an option to export the presentation as a PDF and save it to Your Drive or sent it in an email.
How to implement
First, create a new Google Slides presentation, add the keywords that you want to change with the text from the Form. I suggest adding the fields using the curly brackets {the field}, you can also use double curly brackets.
The next step is to create a Google Form and add the question that you want to update the presentation with.
When you are finished editing the form you must open the script editor and paste the script.
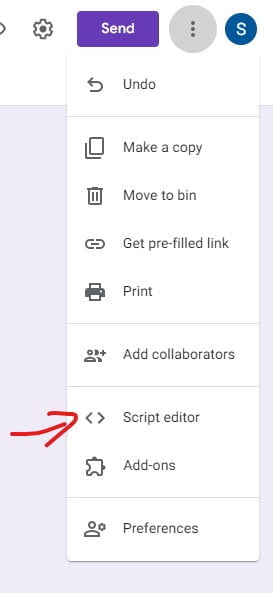
Here is the script:
function onFormSubmit(e) {
//open the template presentation by ID
//you can find the ID in the link of the presentation
var templateDoc = DriveApp.getFileById('1nQwisNPiApKlQ9psmehOC_UQ0Gug7qAq57Id-Sre1CE');
//create a copy of the template, we don't wanna mess up the template presentation
var newTempFile = templateDoc.makeCopy();
//open the presentation for editing
var openSlide = SlidesApp.openById(newTempFile.getId());
//get the responses triggered by On Form Submit
var items = e.response.getItemResponses();
//find the text in the presentation and replace it with the Form response
//items[0].getResponse() is the first response in the Form
//and it is the "Title"
openSlide.replaceAllText('{Title}', items[0].getResponse());
//items[1].getResponse() is the second and it is the date
openSlide.replaceAllText('{Text}', items[1].getResponse());
//You can add as much as you have and change them in the Template Doc like this
//openSlide.replaceAllText('{number}', items[2].getResponse());
//openSlide.replaceAllText('{choice}', items[3].getResponse());
//and so on...
//Save and Close the open document
openSlide.saveAndClose();
DriveApp.getFileById(newTempFile.getId()).setName(items[0].getResponse());
// You can also convert the presentation to PDF and save it your Drive
// or send it in email
// To do that remove block comments on line 34 and 67
/*
var theBlob = newTempFile.getBlob().getAs('application/pdf');
//The name of the file is going to be the first question from the form
//change to your preference
var nameFile = items[0].getResponse() + '.pdf';
//send an email with the PDF
//If you don't want to send the PDF in the mail just delete everything
//from here -------
var email = items[2].getResponse();
var subject = 'Your new documnet';
var body = 'Hello, <br/>Check this PDF file.';
GmailApp.sendEmail(email, subject, body, {
htmlBody: body,
attachments: [{
fileName: nameFile,
content: theBlob.getBytes(),
mimeType: "application/pdf"
}]
});
//to here ------
// save the PDF file in your Drive
var savePDF = DriveApp.createFile (theBlob);
//if you want to save the file in a specific folder use this code
//get the ID from the folder link
//var folder = DriveApp.getFolderById('14nUc----------0lUb');
//var savePDF = folder.createFile (theBlob);
savePDF.setName(nameFile);
*/
}
What to change
First, you need to get the ID of the Google Slides Presentation and replace it in the script on line 4. Here is an image on how to locate the ID of any Google document:
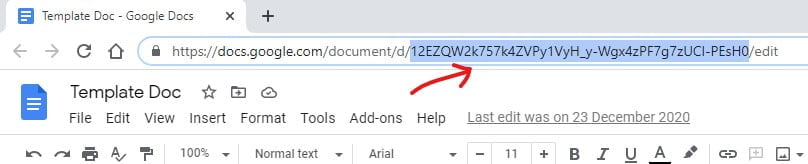
Next, you have to adjust the fields that you need to change with the fields in the form. These commands are located on lines 17 and 19. here are the commands:
openSlide.replaceAllText('{Title}', items[0].getResponse());
openSlide.replaceAllText('{Text}', items[1].getResponse());
This commands tells google to find all the words the are {Title} and change them with the word from the first question in the Form. The second command is used to find and change all the words that are {Text} to the second question in the Form.
You can continue to add this commands to replace more words with your Form questions.
There is more explanation on this method in this article: Google Form to PDF (save to Drive and/or send it in email)
Run the script once just to accept the permission. If you have any problems, check this guide: Google Script Authorization: Review and Accept the Permission Guide.
Add a trigger
In order for the script to execute every time you fill in the form, you must set up a script trigger “on Form Submit”. To do that, click on the “Triggers” from the left-hand menu, click on the “+ Add Trigger” button located in the bottom right corner, In the next window select event type to be “On form submit” and click Save.
That’s it, you can now test the script with submitting a Google Form response.
Export the Google Slide presentation to PDF and save and/or send it in email
The script contains code to export the presentation to PDF and save it to your Drive and send it in email. The part of the script is commented out, so in order to work, you have to remove the comment-out text located on lines 34 and 67.
Now the code between those lines will execute and it will save the exported PDF file in your Drive and it will send an email to the email address summited from the form as a third question. Here is the command, located on line 44:
var email = items[2].getResponse();
If you want to send it only to one email address, you can edit this line and add your email address like this:
var email = 'your@email.address';
That’s it, there are some more explanations on how to customize the script itself, and if you need more info just send in a comment.